Linux Debuggers
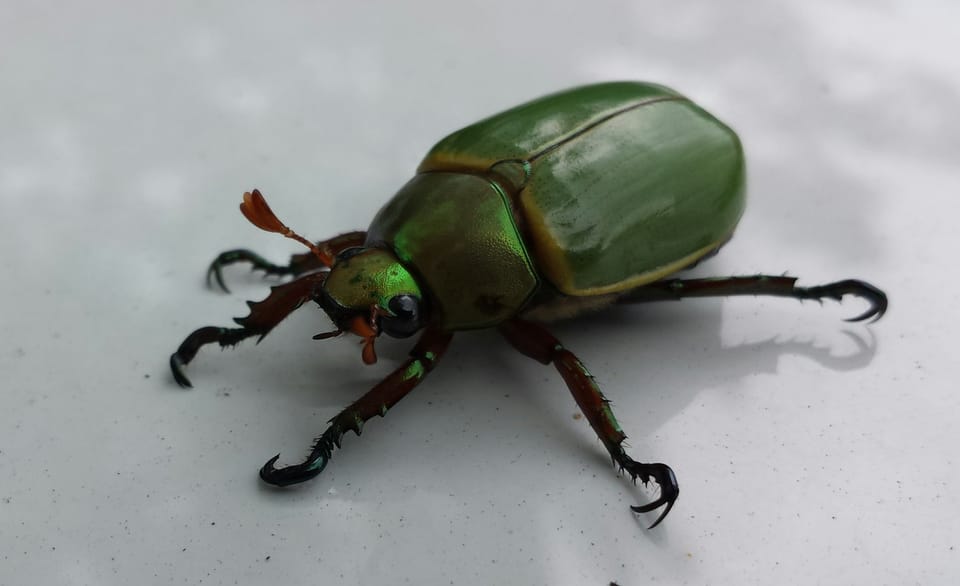
Debuggers
A debugger or debugging tool is a computer program that is used to test and debug other programs (the target program).
Source: Wikipedia
The typical functionalities of a debugger include:
- a query processor;
- a symbol resolver;
- an expression interpreter;
- single-stepping an executable, either at the source-code level for source-level debuggers or at the machine-code level for low-level debuggers;
- interrupting an executable, possibly depending on certain conditions;
- resuming execution at another different location;
- etc.
A breakpoint is a place in your program where the program stops. You can set conditions on breakpoint to make the debugger stop only in certain cases (conditional breakpoints). A watchpoint (or data breakpoint) is a special breakpoint that stops your program when the value of an expression in memory changes. A catchpoint is a special breakpoint that stops your program when a specific event occurs. This could be something like a C++ exception being thrown or a dynamic library being loaded.
Most CPUs offer hardware support for single-stepping (a process where you pause execution at a specific point in a program) and setting code and data breakpoints (where you can pause execution at a specific address or data location). As an illustration, let’s take a look at Intel's debug registers, which are special resources that only ring 0 can access.
DR0
-DR3
: each of these registers contains a linear address associated with one of four breakpoints conditions;DR6
is the debug status register;DR7
is the debug control register.
However, user-land program debugging is usually implemented thanks to software breakpoints. In a nutshell, it consists of replacing the target instruction with an instruction that triggers a software interrupt, which allows the debugger to take control of the debugged program.
Standard debuggers include gdb
(GNU debugger), lldb
, maintained by the LLVM project and that is the Apple ecosystem debugger, and Microsoft's WinDbg
debugger.
Linux ptrace() System Call
In Linux, there's a system call specifically for debugging purposes called ptrace()
. It lets one process control the execution of another process and examine its data. Here is its prototype:
#include <sys/ptrace.h>
long ptrace (enum __ptrace_request request,
pid_t pid,
void *addr,
void *data);
The parameter request
allows to set one of the many operations of ptrace()
. pid
allows to specify the ID of the task (process/thread) on which to perform the operation. addr
is an optional address in tracee's memory that is used for specific operations, like reading or writing and data
is an optional address in tracer's memory for reading and writing to and from the traced task. ptrace()
returns a 0
for success, -1
in case of error, or a word in case of a read operation.
A typical use case of how the ptrace()
system call works is as follows: the ptrace()
system call can be used by a process (the parent/tracer) on a task (the child/tracee) in two ways: by attaching to it or by forking it. On the other hand, a child can stop itself or be stopped by its parent. A task stops when it receives the SIGSTOP
signal or, if it is traced, when it receives a signal (SIGKILL
is an exception). The parent is notified by the wait()
system call when a child is stopped.
Specifically, a standard debugging cycle looks like this: (1) the child runs; (2) the child stops after receiving a signal; (3) the parent receives the child's signal status thanks to wait()
; (4) the parent performs various operations on the stopped child; (5) the parent signals the child that it can continue running.
Here is a non-exhaustive list of operations that can be done with ptrace()
(see man ptrace
for the full documentation):
PTRACE_TRACEME
: a (future) tracee announces to its parent that it wants to be traced. As soon as the tracee makes a call to a member of the exec()
family, it will be stopped until the tracer allows it to continue.
long ret = ptrace (PTRACE_TRACEME, 0, NULL, NULL);
PTRACE_{GETREGS/SETREGS}
: a task wants to get/set the general-purpose registers of the tracee.
#include <sys/user.h>
user_regs_struct regs;
long ret = ptrace (PTRACE_GETREGS, pid, NULL, ®s);
long ret = ptrace (PTRACE_SETREGS, pid, NULL, ®s);
PTRACE_POKEDATA
: a task wants to write data val
in tracee's memory space at address addr
.
long ret = ptrace (PTRACE_POKEDATA, pid, addr, val);
PTRACE_PEEKDATA
: a task wants to read data in tracee's memory space at address addr
.
long word = ptrace (PTRACE_PEEKDATA, pid, addr, NULL);
PTRACE_CONTINUE
: a task wants a traced task to continue (if data == 0
ordata == SIGSTOP
) or to receive a signal (if data == <SIGNAL ID>
).
long ret = ptrace (PTRACE_CONT, target_pid, NULL, 0);
PTRACE_DETACH
: a task wants to stop tracing another task.
long ret = ptrace (PTRACE_DETACH, pid, NULL, NULL);
PTRACE_ATTACH:
A task wants to trace another task. It sends a SIGSTOP
to the child, which should be acknowledged by wait()
on the parent side.
long ret = ptrace (PTRACE_ATTACH, pid, NULL, NULL);
The ptrace()
system calls therefore allow you to implement a debugger, trace system calls, inject code into a running process, check the integrity of a running process, or even implement anti-debugging tricks.
You should be aware that using ptrace()
can potentially cause security problems. The way it works can be changed by setting the /proc/sys/kernel/yama/ptrace_scope
value on certain Linux versions:
The sysctl settings (writable only with CAP_SYS_PTRACE) are:
0 - classic ptrace permissions: a process can PTRACE_ATTACH to any other
process running under the same uid, as long as it is dumpable (i.e.
did not transition uids, start privileged, or have called
prctl(PR_SET_DUMPABLE...) already). Similarly, PTRACE_TRACEME is
unchanged.
1 - restricted ptrace: a process must have a predefined relationship
with the inferior it wants to call PTRACE_ATTACH on. By default,
this relationship is that of only its descendants when the above
classic criteria is also met. To change the relationship, an
inferior can call prctl(PR_SET_PTRACER, debugger, ...) to declare
an allowed debugger PID to call PTRACE_ATTACH on the inferior.
Using PTRACE_TRACEME is unchanged.
2 - admin-only attach: only processes with CAP_SYS_PTRACE may use ptrace
with PTRACE_ATTACH, or through children calling PTRACE_TRACEME.
3 - no attach: no processes may use ptrace with PTRACE_ATTACH nor via
PTRACE_TRACEME. Once set, this sysctl value cannot be changed.
Typically, the default value on Ubuntu distributions is 1
.
For more information about ptrace()
see Pradeep Padala’s articles Playing with ptrace(), Part I, and Playing with ptrace(), Part II, Linux Journal, 2002.
In the next episode, I’ll discuss how to hook calls to shared libraries on Linux. Stay tuned!
Thanks for reading Crumbs of Cybersecurity! Subscribe for free to receive new posts and support my work.