Hooking on Linux
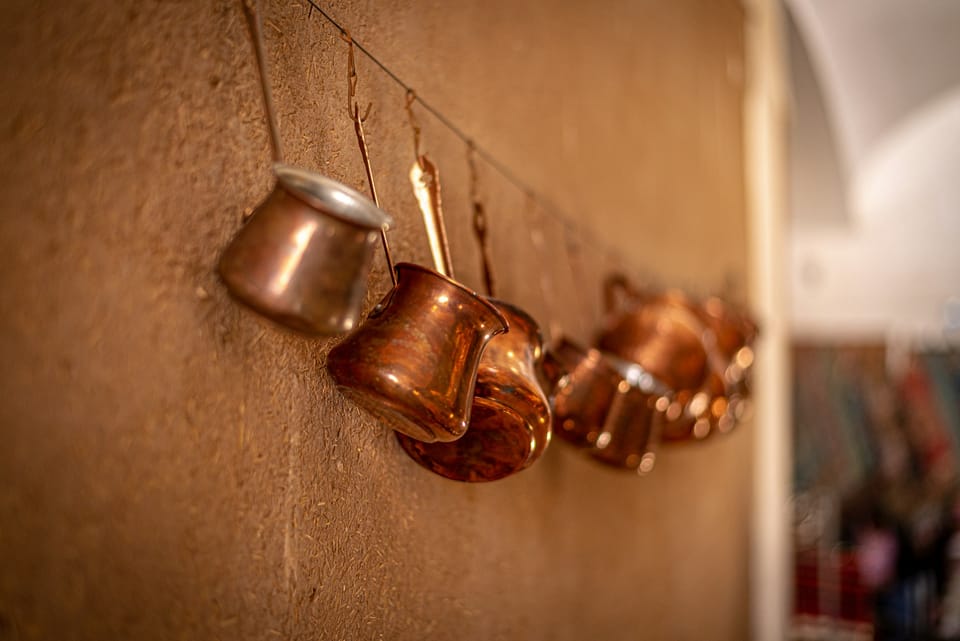
Hooking Shared Libraries
Sometimes, it's useful to hook calls to shared libraries. On Linux, you can do this by using the LD_PRELOAD
environment variable. Let's look at an example to understand this better.
Here is a toy C code:
#include <stdlib.h>
#include <stdio.h>
#include <time.h>
int main(void)
{
int i;
srand(time (NULL));
for (i = 0; i < 10; i++) {
fprintf (stdout, "\nHere is a random number: %d", rand());
}
fprintf(stdout, "\n\n");
return 0;
}
You can compile and run it:
$ gcc -o main main.c
$ ./main
Here is a random number: 1503854890
Here is a random number: 447779094
Here is a random number: 68302234
Here is a random number: 511246255
Here is a random number: 715622854
Here is a random number: 344404168
Here is a random number: 366897690
Here is a random number: 369159294
Here is a random number: 1619623515
Here is a random number: 1340549311
Suppose we want to replace the call to libc
's rand()
function with one that returns a constant value:
int rand()
{
return 0xDEADBEEF; /* Generated using a TRNG for sure */
}
You can create a shared library that exports this new flavor of rand()
, and ask the dynamic linker to load this shared library before loading the others thanks to the LD_PRELOAD
environment variable. As a result, libc
's rand()
will be ignored, since a rand()
symbol is already defined when libc
is loaded.
$ gcc -shared -o better_rand.so -fpic better_rand.c
$ LD_PRELOAD=./better_rand.so ./main
Here is a random number: -559038737
Here is a random number: -559038737
Here is a random number: -559038737
Here is a random number: -559038737
Here is a random number: -559038737
Here is a random number: -559038737
Here is a random number: -559038737
Here is a random number: -559038737
Here is a random number: -559038737
Here is a random number: -559038737
The goals of hooking shared libraries are multiple: testing, bug patching, removing software protections, application hacking, etc.
Hooking with Frida
The Frida Toolkit is a free and open source dynamic instrumentation toolkit. It allows you to insert custom JavaScript code into an application and supports Windows, macOS, Linux, Android, iOS, and QNX operating systems. It is particularly popular for hacking mobile applications.
Frida's core is written in C and injects a JavaScript (JS) engine into the target process. Custom JavaScript (JS) scripts can be executed with full memory access, hooking and calling the internal functions of the process. It is typically operated by Python scripts, but bindings for other languages are available.
Let me go back to our toy example and write a Frida hook in Python that targets the rand()
symbol:
$ pip3 install frida-tools
$ frida-trace -i "rand" ./main
Instrumenting...
rand: Auto-generated handler at "/tmp/frida_example/__handlers__/libc_2.31.so/rand.js"
Here is a random number: 683265480
Here is a random number: 1674476993
Here is a random number: 1799471881
Here is a random number: 1411106989
...
Started tracing 1 function. Press Ctrl+C to stop.
/* TID 0x52e7 */
242 ms rand()
242 ms rand()
242 ms rand()
...
You can have a look at the auto-generated …/rand.js file:
// ...
onEnter(log, args, state) {
log('rand()');
},
// ...
onLeave(log, retval, state) {
}
// ...
Two callbacks have been automatically defined to be executed just before and just after calls to rand()
. It is very easy to replace the value returned by rand()
by adding a line to the onLeave()
callback:
// ...
onEnter(log, args, state) {
log('rand()');
},
// ...
onLeave(log, retval, state) {
retval.replace(1337);
}
// ...
Running the program a new time results in:
$ frida-trace -i "rand" ./main
Instrumenting...
rand: Loaded handler at "/tmp/frida_example/__handlers__/libc_2.31.so/rand.js"
Here is a random number: 1337
Here is a random number: 1337
Here is a random number: 1337
...
In the next episode, I’ll discuss binary instrumentation. Stay tuned!
Thanks for reading Crumbs of Cybersecurity! Subscribe for free to receive new posts and support my work.