Binary Instrumentation
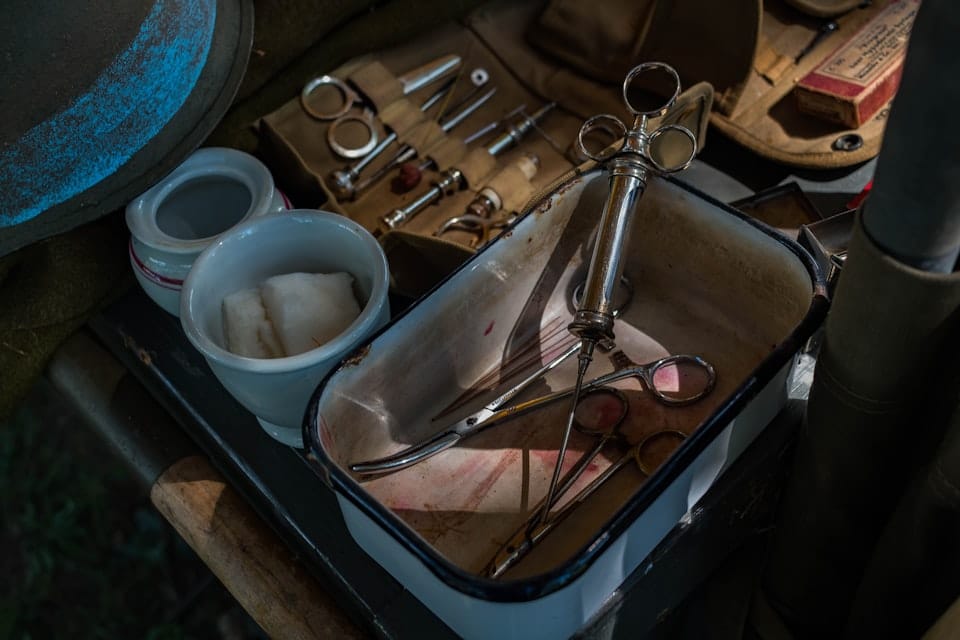
Basics
In the context of computer programming, instrumentation refers to the measure of a product's performance, to diagnose errors, and to write trace information. Instrumentation can be of two types: source instrumentation and binary instrumentation.
Source: Wikipedia
Instrumentation is the ability of an application to incorporate the following:
- tracing, that is receiving informative messages about the execution of an application at runtime;
- debugging, to find and fix programming errors in an application under development;
- profiling, which is a means of measuring dynamic program behavior during a training run with a representative input.
A typical example is profiling the performances of a program with GNU tools. It consists of three steps:
- Add profiling code at the compilation level, for example using the
-pg
option ofgcc
. - Running the profiled application to generate profiling data.
- Analyze the data using gprof.
Another example are the dynamic analysis capabilities of the LLVM Clang compiler:
- AddressSanitizer (option
-fsanitize=address
): it allows to reveal out-of-bounds accesses to heap, stack and globals, use-after-free, use-after-return, double-free, invalid free, or memory leaks. - ThreadSanitizer (option
-fsanitize=thread
): it allows to detect data races. - MemorySanitizer (option
-fsanitize=memory
): it is a detector of uninitialized memory reads. - LeakSanitizer (option
-fsanitize=leak
}): it is a run-time memory leak detector. - and other tools such as the UndefinedBehaviourSanitizer, the DataFlowSanitizer, etc.
Another example of a tool that relies on code instrumentation is Valgrind. It is essentially a virtual machine that uses just-in-time (JIT) compilation techniques, including dynamic recompilation. Valgrind first translates the program into a temporary, simpler form called an intermediate representation (IR). The IR has a processor-neutral, SSA-based form. After the conversion, a tool can make any changes it wants to the IR. Then Valgrind translates the IR back into machine code, and the host processor executes it.
Valgrind's most popular tool is memcheck
. It finds problems such as using uninitialized memory, reading or writing memory after it has been freed, reading or writing off the end of malloc'd blocks, and memory leaks. There are also other tools for performance analysis and profiling.
The Pin Framework
Pin is a free, but closed source binary instrumentation framework developed by Intel Corp. Pin supports the Linux, (macOS) and Microsoft Windows operating systems and executables for the 32- and 64-bit Intel architectures.
Pin allows you to insert any code (written in C or C++) at any location in the executable. The code is dynamically added while the executable is running. You can also attach Pin to an already running process.
One could interpret Pin as a just in time (JIT) compiler, whose input is not bytecode, but a regular executable. Pin intercepts the execution of the first instruction of the executable and generates (compiles) new code for the basic block code starting from that instruction.
The generated code is almost identical to the original one, but Pin ensures that it regains control when a branch leaves the basic block. After regaining control, Pin generates more code for the branch target and continues execution. Pin instruments all instructions that are actually executed, regardless of where they are.
Binary instrumentation has two parts. First, there is a mechanism that decides where and what code to insert, called the instrumentation code; second there is the code to execute at insertion points called the analysis code. Both parts make up a pintool. Pintools can be thought of as plugins that can modify the code generation process within Pin.
Concretely, a pintool is a tool that registers callback routines with Pin. The routines are called from Pin whenever new code needs to be generated. They form the instrumentation part. This part checks the code that needs to be generated. It also looks at its static properties. Finally, it decides where to inject calls to analysis functions. The analysis part gathers data about the application. The pintool can also register notification callback routines for events such as thread creation, forking, and system calls.
The best way to get familiar with the Pin framework and its API is to read the source code and build the many examples that are provided:
# On a 32-bit architecture:
$ cd source/tools/ManualExamples
$ make all TARGET=ia32
# On a 64-bit architecture:
$ cd source/tools/ManualExamples
$ make all TARGET=intel64
One of the simplest example is a pintool allowing to counter the number of machine instructions executed by a program:
#include <iostream>
#include <fstream>
#include "pin.H"
ofstream OutFile;
// The running count of instructions is kept here
// make it static to help the compiler optimize docount
static UINT64 icount = 0;
// This function is called before every
// instruction is executed
VOID docount() { icount++; }
// Pin calls this function every time a new instruction
// is encountered
VOID Instruction(INS ins, VOID *v)
{
// Insert a call to docount before every
// instruction, no arguments are passed
INS_InsertCall(ins, IPOINT_BEFORE, (AFUNPTR)docount, IARG_END);
}
// This function is called when the application exits
VOID Fini(INT32 code, VOID *v)
{
// Write to a file since cout and cerr may be
// closed by the application
OutFile.setf(ios::showbase);
OutFile << "Count " << icount << endl;
OutFile.close();
}
INT32 Usage()
{
cerr << "This tool counts the number of ";
cerr << "dynamic instructions executed" << endl;
cerr << endl << KNOB_BASE::StringKnobSummary() << endl;
return -1;
}
KNOB<string> KnobOutputFile(KNOB_MODE_WRITEONCE, "pintool",
"o", "inscount.out", "specify output file name");
int main(int argc, char * argv[])
{
if (PIN_Init(argc, argv)) return Usage();
OutFile.open(KnobOutputFile.Value().c_str());
// Register Instruction to be called to instrument instructions
INS_AddInstrumentFunction(Instruction, 0);
// Register Fini to be called when the application exits
PIN_AddFiniFunction(Fini, 0);
// Start the program, never returns
PIN_StartProgram();
return 0;
}
Here, docount()
is the analysis part: it is called each time an instruction is executed and increments the global counter icount
. The instrumentation part is represented by the Instruction()
function, whose task is to insert a call to docount()
before every instruction executed by the program.
Pintools can be very powerful and flexible tools, but they come with significant performance challenges.
In the next episode, I’ll discuss the basics of software protection. Stay tuned!
Thanks for reading Crumbs of Cybersecurity! Subscribe for free to receive new posts and support my work.