Basics of the Intel Architecture
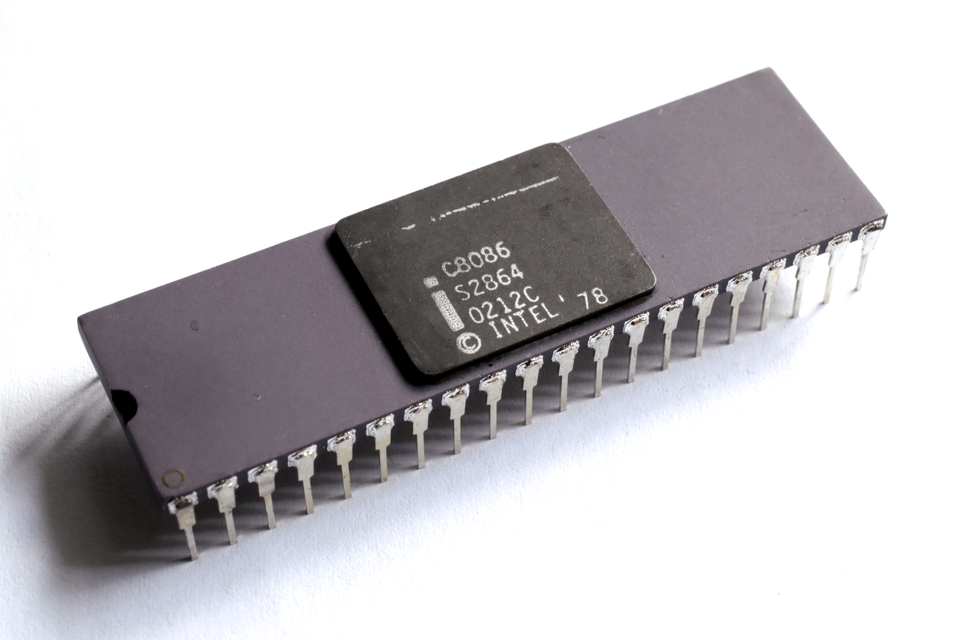
Intel Architecture
The Intel architecture is a little-endian, Complex Instruction Set Computer (CISC) architecture. The 32-bit version is called x86
or IA-32, while the 64-bit version is called x64
or x86-64
; don't confuse it with the discontinued IA-64 (Itanium) architecture.
A comprehensive documentation of the architecture is available in the Intel 64 and IA-32 architectures software developer's manual (4800+ pages).
x86 supports several operating modes, including real mode, protected mode and long mode. While real mode provides no support for memory protection, multitasking, or code privilege levels, it is still the default mode when an Intel CPU is reset.
In protected and long modes, the concept of privilege separation is supported by rings ranging from ring 0 to ring 3. Ring 0 represents the highest privilege level, with the ability to modify all system settings, typically referred to as kernel land. Ring 3 represents the lowest privilege level, with the ability to read and modify only a subset of system settings, typically referred to as user land.
Registers
In 32-bit mode, there are 8 general-purpose registers: EAX
, EBX
, ECX
, EDX
, EDI
, ESI
, EBP
, and ESP
. In 64-bit mode, there are 16 general-purpose registers: RAX
, RBX
, RCX
, RDX
, RDI
, RSI
, RBP
, RSP
, and R8
through R15
.
Lower bits of a register can be directly accessed by opcodes: for instance, the 32 lower bits of the 64-bit register RAX
are named EAX
; it 16 lower bits are named AX
, which itself is split in AH
(bits 15 to 8) and AL
(bits 7 to 0).
The instruction register EIP
/RIP
contains the address of the next instruction to be executed.
With certain machine instructions, some of these registers have special purposes: ECX
/RCX
are used as counters in loops, ESI
/RSI
stores the source address in string/memory operations while EDI
/RDI
stores the destination address, EBP
/RBP
stores the base frame pointer, and ESP
/RSP
contains the stack pointer.
Depending on the architecture version, other sets of general-purpose registers are available, such as floating-point registers, or SIMD registers.
The x86
instruction set allows a variety of data movements:
- immediate value (constant) to register;
- register to register;
- immediate value to memory;
- register to memory;
- memory to register;
- memory to memory.
Intel and AT&T Syntaxes
Depending on the assembler or the disassembler, there are two different ways to write x86 assembly code: Intel syntax and AT&T syntax. Microsoft tools on Windows usually use the Intel notation. AT&T is more common on Unix and similar operating systems.
; Intel syntax
mov ecx, DEADBEEFh
mov ecx, [eax]
mov eax, ecx
; AT&T syntax
movl $0xDEADBEEF, %ecx
movl (%eax), %ecx
movl %ecx, %eax
The main difference are as follows:
- AT&T puts the source operand before the destination operand. Intel does the opposite.
- AT&T prefixes the register with
%
and constants (immediates) with$
. - AT&T adds a suffix to the instruction to indicate the operation width (
MOVL
vs.MOVB
). Here,MOVL
refers to moving a long word, whileMOVB
refers to moving a byte.
Instructions
There are hundreds of instructions in the Intel architecture. The main categories are:
- Data moving instructions:
MOV
,MOVSB
,MOVSW
,LEA
, … - Arithmetic and Boolean instructions:
ADD
,SUB
,MUL
,DIV
,INC
,NEG
,AND
,OR
,XOR
,NOT
, … - Strings-related instructions:
SCAS
,STOS
, … - Stack- and subroutine-related instructions:
PUSH
,POP
,CALL
,RET
, … - Control-flow-related instructions:
CMP
,TEST
,JMP
,Jcc
, … - in addition to system-, vector-, security-related instructions, etc.
Control Flow Modification
The instruction control flow can be modified through instructions such as CMP
, TEST
, JMP
, and Jcc
instructions as well as the EFLAGS/RFLAGS register which stores the following flag bits, among others:
ZF
(zero flag): set if the result of the previous arithmetic operation is zero.SF
(sign flag): set to the most significant bit of the result.CF
(carry flag): set when the result requires a carry. It applies to unsigned integers.OF
(overflow flag): set if the result overflows the maximal size. It applies to signed integers.
The CMP
instruction compares two operands by subtracting them and sets the appropriate flags. The TEST
instruction compares two operands by computing a Boolean AND
and sets the appropriate flags. JMP
is an unconditional branch operation.
Jcc
is a conditional branch operation based on the condition code cc
. The main condition codes are:
B
/NAE
(unsigned operations): below/neither above nor equal (CF == 1
)NB
/AE
(unsigned operations): not below/above or equal (CF == 0
)E
/Z
: equal/zero (ZF == 1
)NE
/NZ
: not equal/non-zero (ZF == 0
)L
(signed operations): less than/neither greater nor equal (SF^OF == 1
)GE
/NL
(signed operations): greater or equal/not less than(SF^OF == 0
)G
/NLE
(signed operations): greater/not less nor equal((SF^OF)|ZF == 0
)
As an illustration, let us have a look at the following C code:
#include <stdio.h>
int main(void)
{
unsigned int i = 0xDEADBEEFUL;
if (i > 12) { fprintf(stdout, "\ni > 12"); } else { fprintf(stdout, "\ni <= 12"); }
while (i >= 12) { i--; }
switch (i) {
case 12:
fprintf(stdout, "\ni == 12");
break;
case 11:
fprintf(stdout, "\ni == 11");
goto label;
default:
fprintf(stdout, "\ni != 12 and i != 11");
}
label:
return 0;
}
The IDA Pro disassembler produces the following result:
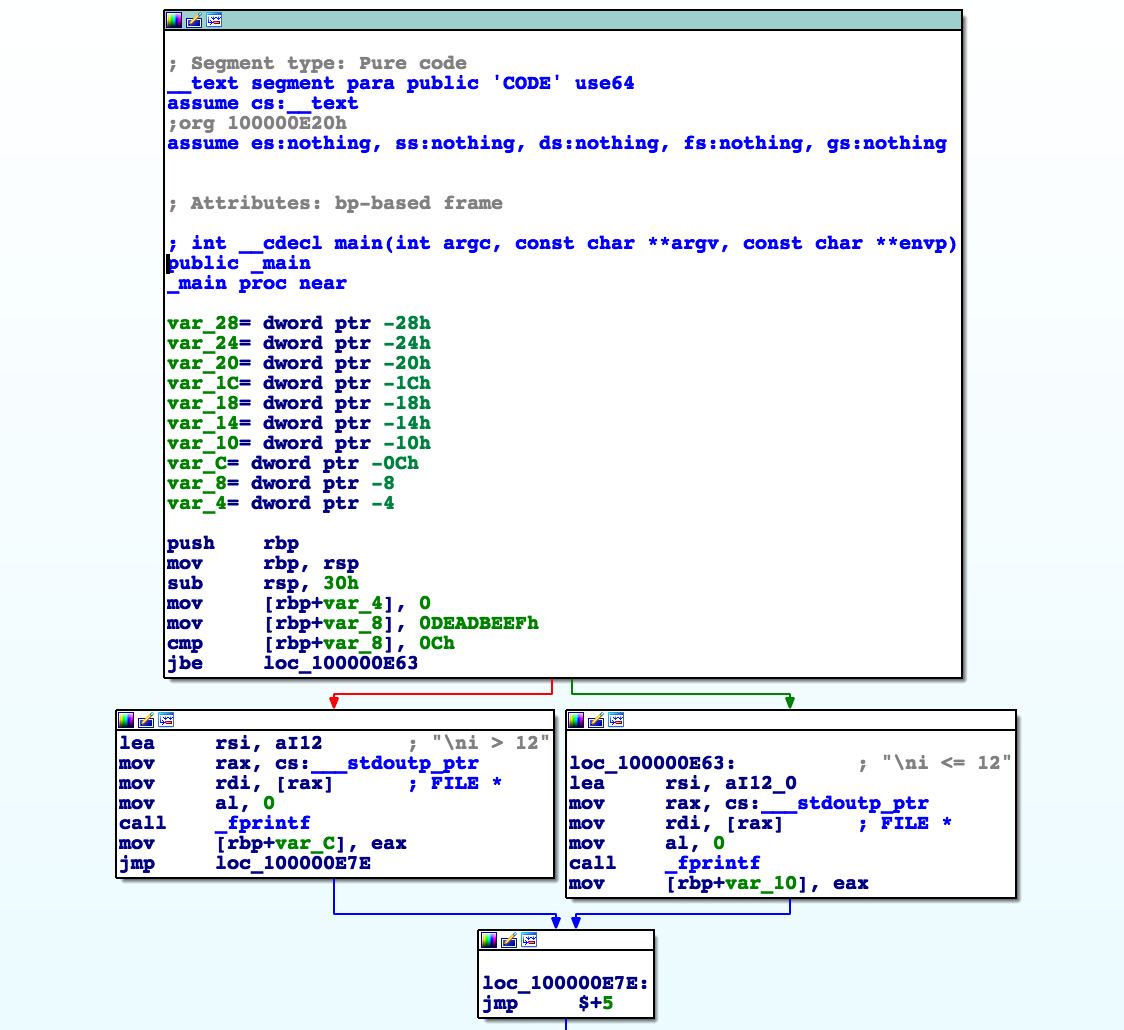
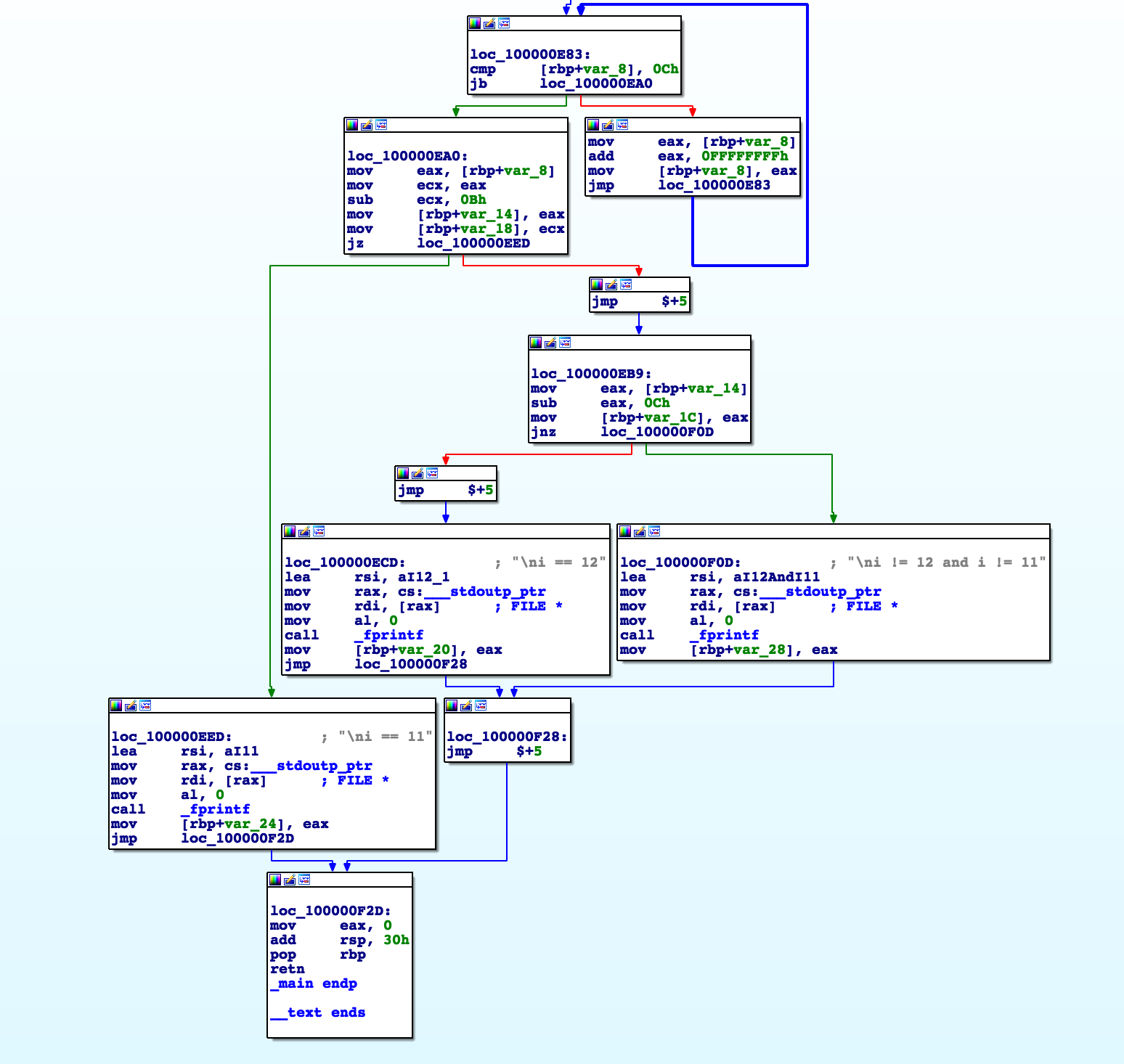
You can see how instructions such as JMP
, JB
, JZ
, and JNZ
modify the control flow.
In the next episode, I will cover the basics of the ARM architecture. Stay tuned!
Thanks for reading Crumbs of Cybersecurity! Subscribe for free to receive new posts and support my work.